Ok, I got it!
For those who are interested:
Here’s a shader that can swap unlimited amounts of colors.
You can copy paste it and save as a module to your project.
local shader = {}
local kernel = {}
kernel.language = "glsl"
kernel.category = "filter"
--kernel.group = "custom"
kernel.name = "multiswap"
kernel.uniformData = {
{
name = "keys",
default = {
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0
},
min = {
0.0, 0.0, 0.0, 0.0,
0.0, 0.0, 0.0, 0.0,
0.0, 0.0, 0.0, 0.0,
0.0, 0.0, 0.0, 0.0
},
max = {
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0
},
type="mat4",
index = 0
},
{
name = "colors",
default = {
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0
},
min = {
0.0, 0.0, 0.0, 0.0,
0.0, 0.0, 0.0, 0.0,
0.0, 0.0, 0.0, 0.0,
0.0, 0.0, 0.0, 0.0
},
max = {
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0,
1.0, 1.0, 1.0, 1.0
},
type="mat4",
index = 1
}
}
kernel.fragment = [[
uniform P_COLOR mat4 u_UserData0; // keys
uniform P_COLOR mat4 u_UserData1; // colors
P_COLOR vec4 FragmentKernel( P_UV vec2 texCoord )
{
P_COLOR vec4 texColor = texture2D( CoronaSampler0, texCoord );
for(int i = 0; i < 50; i++)
{
P_COLOR vec4 keys = u_UserData0[i];
P_COLOR vec4 colors = u_UserData1[i];
if ((abs(texColor[0] - keys[0]) < 0.01) && (abs(texColor[1] - keys[1]) < 0.01) && (abs(texColor[2] - keys[2]) < 0.01))
{
texColor = colors;
break;
}
}
return CoronaColorScale(texColor);
}
]]
graphics.defineEffect( kernel )
shader.swap = function( object, fromColors, toColors )
local keys = {}
local colors = {}
for i=1,#fromColors do
for ii=1,4 do
if ii < 4 then
table.insert(keys, fromColors[i][ii]/255)
table.insert(colors, toColors[i][ii]/255)
else
table.insert(keys, 1)
table.insert(colors, 1)
end
end
end
object.fill.effect = "filter.custom.multiswap"
object.fill.effect.keys = keys
object.fill.effect.colors = colors
end
return shader
This is how you use it:
Just create 2 tables with the colors you want to change.
In this example we swap 3 colors.
local shader = require "shader"
local fromColor = {
{ 223, 223, 223 },
{ 191, 191, 191 },
{ 147, 147, 147 }
}
local toColor = {
{ 243, 196, 163 },
{ 202, 117, 101 },
{ 96, 64, 62 }
}
shader.swap( YOUR-OBJECT, fromColor, toColor )
The color codes are RGB, you get from software like photoshop.
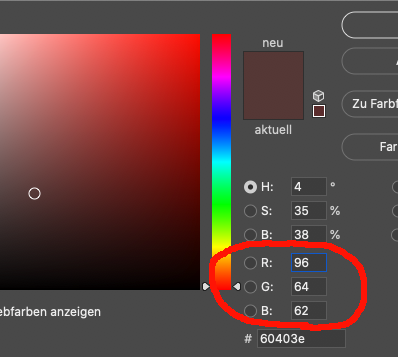
NOTE:
Right now the module can swap 50 colors.
If you want to have more, just adjust the value within the modules for-loop:
Hope that helps someone.